今回は、Pythonを使って特定のWebページが更新された際に、1時間ごとに自動でチェックし、LINEまたはメールで通知を送る方法を紹介します。
不動産の情報を仕入れる場合はポータルサイトより前に自社のサイトに掲載されますが更新を知らせる
必要な準備
- Python 3.x
requests
ライブラリbeautifulsoup4
ライブラリ- 通知方法として、LINE Notify または メール を使用
LINE Notifyの準備
- LINE Notifyにアクセスし、アクセストークンを取得します。
- 通知を送りたいLINEアカウントと連携し、アクセストークンを取得します。
メール通知の準備
メール通知を利用するには、SMTPサーバーが必要です。Gmailを使って送信する場合、以下の情報が必要です。
- SMTPサーバー:
smtp.gmail.com
- ポート: 587
- Gmailアドレスとアプリパスワード
Pythonコード: Webページ更新チェックとLINE/メール通知
次のPythonコードでは、1時間ごとにWebページをチェックし、LINEまたはメールで通知を送る仕組みを実装しています。
import requests
import os
import time
from bs4 import BeautifulSoup
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# LINE Notifyのアクセストークン
LINE_NOTIFY_TOKEN = "YOUR_LINE_NOTIFY_TOKEN"
# メール送信用の設定
FROM_EMAIL = "your_email@gmail.com"
EMAIL_PASSWORD = "your_app_password"
TO_EMAIL = "recipient_email@example.com"
# WebページのURLリスト
urls = [
"https://example1.com",
"https://example2.com",
"https://example3.com"
]
# ページのHTMLを保存するディレクトリ
content_dir = "page_contents"
# BeautifulSoupでページの内容を取得する関数
def fetch_page_content(url):
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
return soup.prettify()
else:
print(f"Error {response.status_code} fetching {url}")
return None
# ページの内容が変更されたか確認する関数
def has_page_changed(new_content, file_path):
if not os.path.exists(file_path):
return True
with open(file_path, 'r', encoding='utf-8') as file:
previous_content = file.read()
return new_content != previous_content
# ページの内容を保存する関数
def save_page_content(content, file_path):
with open(file_path, 'w', encoding='utf-8') as file:
file.write(content)
# LINE Notifyで通知を送る関数
def send_line_notify(message):
url = "https://notify-api.line.me/api/notify"
headers = {"Authorization": f"Bearer {LINE_NOTIFY_TOKEN}"}
data = {"message": message}
requests.post(url, headers=headers, data=data)
# メールで通知を送る関数
def send_email(subject, message):
msg = MIMEMultipart()
msg['From'] = FROM_EMAIL
msg['To'] = TO_EMAIL
msg['Subject'] = subject
body = MIMEText(message, 'plain')
msg.attach(body)
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(FROM_EMAIL, EMAIL_PASSWORD)
server.sendmail(FROM_EMAIL, TO_EMAIL, msg.as_string())
server.quit()
# メイン処理
def main():
if not os.path.exists(content_dir):
os.makedirs(content_dir)
changed_urls = []
for url in urls:
file_name = os.path.join(content_dir, url.replace("https://", "").replace("/", "_") + ".html")
new_content = fetch_page_content(url)
if new_content is None:
continue
if has_page_changed(new_content, file_name):
changed_urls.append(url)
save_page_content(new_content, file_name)
if changed_urls:
message = "以下のWebページが更新されました:\n" + "\n".join(changed_urls)
send_line_notify(message) # LINE Notifyで通知
send_email("Webページ更新通知", message) # メールで通知
else:
print("変更はありませんでした")
if __name__ == "__main__":
while True:
main() # メイン処理を実行
time.sleep(3600) # 1時間(3600秒)待機
スクリプトの実行と定期実行設定
このスクリプトを実行するためには、Python環境が動作している状態で、次のコマンドでスクリプトを起動します。
python your_script.py
スクリプトは1時間ごとに自動的にWebページを確認し、更新があれば通知を送ります。
通知が不要の場合はsend_line_notify(message)とsend_email(“Webページ更新通知”, message)を削除してください。
上記を実行することでWebページに変化があればラインかメールで通知がきます。
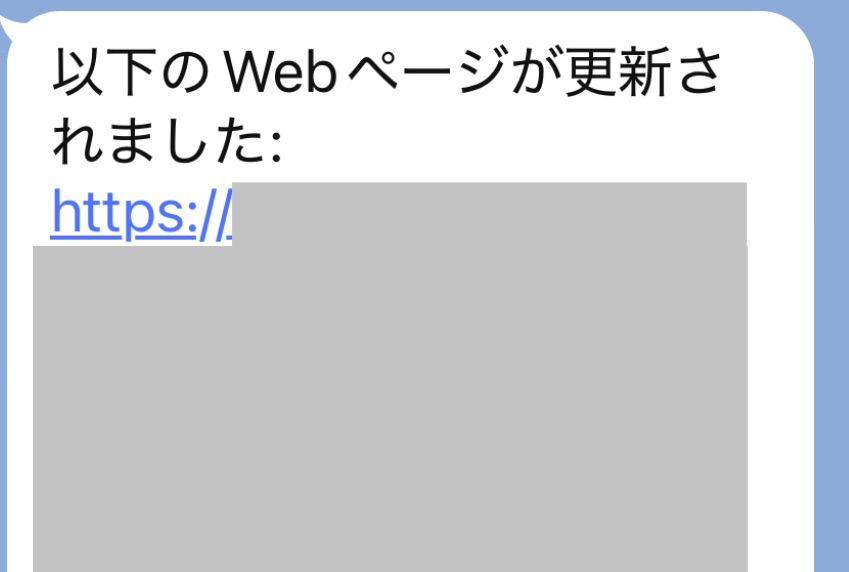
注意点:更新の頻度は1時間に1回としていますが短時間で更新するとサーバーに負担がかかりアクセスの制限をされる恐れがあるため間隔は長めにとるようにしてください。
コメント